Developer Guide
- Acknowledgements
- Setting up, getting started
- Design
- Implementation
- Documentation, logging, testing, configuration, dev-ops
- Appendix: Requirements
-
Appendix: Instructions for manual testing
- Launch and shutdown
- Adding a customer/employee/supplier
- Adding a reservation
- Listing customers/employees/suppliers/reservations
- Deleting a customer/employee/supplier/reservation
- Editing a customer
- Editing a supplier
- Editing an employee
- Finding customers
- Finding suppliers
- Finding employees
- Get the corresponding customer of a reservation
- Get the corresponding customer of a reservation
- Setting the tables
- Checking for reservations
- Sorting the customer list
- Sorting the employee list
- Sorting the Supplier list
- Resetting the customer/employee/supplier
Acknowledgements
- This project is based on the AddressBook-Level3 project created by the SE-EDU initiative.
- We would like to thank Jun Xiong for the invaluable guidance.
Setting up, getting started
Refer to the guide Setting up and getting started.
Design

.puml
files used to create diagrams in this document can be found in the diagrams folder. Refer to the PlantUML Tutorial at se-edu/guides to learn how to create and edit diagrams.
Architecture
The Architecture Diagram given above explains the high-level design of the App.
Given below is a quick overview of main components and how they interact with each other.
Main components of the architecture
Main
has two classes called Main
and MainApp
. It is responsible for,
- At app launch: Initializes the components in the correct sequence, and connects them up with each other.
- At shut down: Shuts down the components and invokes cleanup methods where necessary.
Commons
represents a collection of classes used by multiple other components.
The rest of the App consists of four components.
-
UI
: The UI of the App. -
Logic
: The command executor. -
Model
: Holds the data of the App in memory. -
Storage
: Reads data from, and writes data to, the hard disk.
How the architecture components interact with each other
The Sequence Diagram below shows how the components interact with each other for the scenario where the user issues the command delete 1
.
Each of the four main components (also shown in the diagram above),
- defines its API in an
interface
with the same name as the Component. - implements its functionality using a concrete
{Component Name}Manager
class (which follows the corresponding APIinterface
mentioned in the previous point.
For example, the Logic
component defines its API in the Logic.java
interface and implements its functionality using the LogicManager.java
class which follows the Logic
interface. Other components interact with a given component through its interface rather than the concrete class (reason: to prevent outside component’s being coupled to the implementation of a component), as illustrated in the (partial) class diagram below.
The sections below give more details of each component.
UI component
The API of this component is specified in Ui.java
The UI consists of a MainWindow
that is made up of parts e.g.CommandBox
, ResultDisplay
, PersonListPanel
, StatusBarFooter
etc. All these, including the MainWindow
, inherit from the abstract UiPart
class which captures the commonalities between classes that represent parts of the visible GUI.
The UI
component uses the JavaFx UI framework. The layout of these UI parts are defined in matching .fxml
files that are in the src/main/resources/view
folder. For example, the layout of the MainWindow
is specified in MainWindow.fxml
The UI
component,
- executes user commands using the
Logic
component. - listens for changes to
Model
data so that the UI can be updated with the modified data. - keeps a reference to the
Logic
component, because theUI
relies on theLogic
to execute commands. - depends on some classes in the
Model
component, as it displaysPerson
object residing in theModel
.
Logic component
API : Logic.java
Here’s a (partial) class diagram of the Logic
component:
How the Logic
component works:
- When
Logic
is called upon to execute a command, it uses theRhrhParser
class to parse the user command. - This results in a
Command
object (more precisely, an object of one of its subclasses e.g.,AddEmployeeCommand
) which is executed by theLogicManager
. - The command can communicate with the
Model
when it is executed (e.g. to add a person). - The result of the command execution is encapsulated as a
CommandResult
object which is returned back fromLogic
.
Here are the other classes in Logic
(omitted from the class diagram above) that are used for parsing a user command:
How the parsing works:
- When called upon to parse a user command, the
RhrhParser
class creates anXYZCommandParser
(XYZ
is a placeholder for the specific command name e.g.,AddSupplierCommandParser
) which uses the other classes shown above to parse the user command and create aXYZCommand
object (e.g.,AddSupplierCommand
) which theRhrhParser
returns back as aCommand
object. - All
XYZCommandParser
classes (e.g.,AddSupplierCommandParser
,DeleteSupplierCommandParser
, …) inherit from theParser
interface so that they can be treated similarly where possible e.g, during testing.
Model component
API : Model.java
The Model
component,
- stores RHRH data i.e., all
Customer
,Employee
,Supplier
andReservation
objects (which are contained in aUniqueCustomerList
,UniqueEmployeeList
,UniqueSupplierList
andUniqueReservationList
object respectively). - stores the currently ‘selected’
Customer
/Employee
/Supplier
/Reservation
objects (e.g., results of a search query) as a separate filtered list which is exposed to outsiders as an unmodifiableObservableList<Customer>
/ObservableList<Employee>
/ObservableList<Supplier>
/ObservableList<Reservation>
respectively that can be ‘observed’ e.g. the UI can be bound to this list so that the UI automatically updates when the data in the list change. - stores a
UserPref
object that represents the user’s preferences. This is exposed to the outside as aReadOnlyUserPref
objects. - does not depend on any of the other three components (as the
Model
represents data entities of the domain, they should make sense on their own without depending on other components)

Tag
list in the Rhrh
, which Person
references. This allows Rhrh
to only require one Tag
object per unique tag, instead of each Person
needing their own Tag
objects.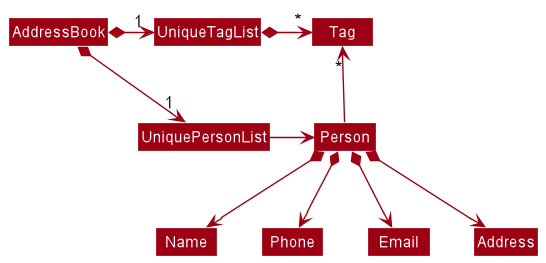
Storage component
API : Storage.java
The Storage
component,
- can save both RHRH data and user preference data in json format, and read them back into corresponding objects.
- inherits from both
RhrhStorage
andUserPrefStorage
, which means it can be treated as either one (if only the functionality of only one is needed). - depends on some classes in the
Model
component (because theStorage
component’s job is to save/retrieve objects that belong to theModel
)
Common classes
Classes used by multiple components are in the seedu.addressbook.commons
package.
Implementation
This section describes some noteworthy details on how certain features are implemented.

Add command
In the following section, we will be going through how our add command works. Since the add command is very similar across all person types, we will be using the AddEmployeeCommand
to illustrate how the add command works. More information on the differences in adding Customer
, Employee
and Supplier
can be found in our User Guide
Current Implementation
Adding an employee can be split into 2 steps: Parse
and Execute
.
Below is a partial class diagram for AddEmployeeCommand
. Note that to make it more comprehensible, details not
related to AddEmployeeCommand
are abstracted away.
The sequence Diagram below illustrates the interactions within the Logic
component for the execute
API call.
Parse
:
- When
Logic
is called upon to executeAddEmployeeCommand
, it uses theRhrhParser
class to parse the user command. - The
RhrhParser
then creates anAddEmployeeCommandParser
to parse the command input. - If successful, this results in an
AddEmployeeCommand
object being created which will then be executed by theLogicManager
.
Execute
:
- The instance of
AddEmployeeCommand
created can communicate with theModel
when it is executed to add an employee to theUniqueEmployeeList
. - The result of the command execution is encapsulated as a
CommandResult
object which is returned back fromLogic
.
Flow of execution
The activity diagram below shows the flow of execution when a user calls the AddEmployeeCommand
together with the
details when this command is executed
Design Considerations
- Current Design: We separated
AddCustomerCommmand
,AddEmployeeCommmand
andAddSupplierCommmand
as different command types.- Pros
- It is much neater as everything is divided based on the Person type and prefixes are easily separated based on whichever Person Type it is.
- Errors in user input is easier to identify due to the separation of Person type.
- Cons
- Additional classes have to be implemented.
- More commands for a similar feature.
- Pros
- Alternative Design: We could have a singular
AddCommand
and parse the user’s input to see what Person Type the user wanted to add.- Pros
- Fewer commands for the user to remember.
- Cons
- Error messages would have been confusing to select.
- Pros
Differences regarding adding reservation command
Parsing Reservecommand
is similar to other add commands. However, since for Reservation
, we need some logic to check for restaurant availability, then there are some differences regarding how a Reservecommand
is executed.
The class diagram below demonstrates the class structure of Reservecommand
.
The sequence diagram below shows how a valid ReserveCommand
is executed.
Edit command
In the following section, we will be going through how our edit command works. Since the edit command is very similar across all person types, we will be using the EditCustomerCommand
to illustrate how the edit command works. More information on the differences in editing Customer
, Employee
and Supplier
can be found in our User Guide
Current Implementation
Editing a customer can be split into 2 steps: Parse
and Execute
.
Below is a partial class diagram for EditCustomerCommand
. Note that to make it more comprehensible, details not
related to EditCustomerCommand
are abstracted away.
The sequence Diagram below illustrates the interactions within the Logic
component for the execute
API call.
Parse
:
- When
Logic
is called upon to executeEditCustomerCommand
, it uses theRhrhParser
class to parse the user command. - The
RhrhParser
then creates anEditCustomerCommandParser
to parse the command input. - If successful, this results in an
EditCustomerCommand
object being created which will then be executed by theLogicManager
.
Execute
:
- The instance of
EditCustomerCommand
created can communicate with theModel
when it is executed to add an customer to theUniqueCustomerList
. - The result of the command execution is encapsulated as a
CommandResult
object which is returned back fromLogic
.
Flow of execution
The activity diagram below shows the flow of execution when a user calls the EditCustomerCommand
together with the
details when this command is executed
Design Considerations
Due to the similarities of the design considerations between this command and the Add
command, please refer to the Design Considerations
section of the Add
command above for more information.
Differences regarding editing reservation command
Implementation of EditReservationcommand
is similar to other edit commands. However, to avoid clashing of the edited reservation with other reservations, only the editing of tags and remark is allowed.
Delete Command
In the following section, we will be going through how our delete command works. Since the delete command is very
similar across all person types, we will be using the DeleteSupplierCommand
to illustrate how the delete
command
works. More information on the differences in deleting Customer
, Employee
and Reservation
can be found in our
User Guide
Current Implementation
Deleting a supplier can be split into 2 steps: Parse
and Execute
.
Here’s a partial class diagram for the DeleteSupplierCommand
. Note that to make it more comprehensible, details
not related to the DeleteSupplierCommand
are abstracted away.
The Sequence Diagram below illustrates the interactions within the Logic
component for the execute("deleteS 1")
API call.
Parse
:
- When
Logic
is called upon to execute a command, it uses theRhrhParser
class to parse the user command. - The
RhrhParser
then creates aDeleteSupplierCommandParser
to parse the command input. - If successful, this results in a
DeleteSupplierCommand
object being created which then is executed by theLogicManager
.
Execute
:
- The instance of
DeleteSupplierCommand
created can communicate with theModel
when it is executed to delete a supplier from theUniqueSupplierList
. - The result of the command execution is encapsulated as a
CommandResult
object which is returned back fromLogic
.
Flow of execution
The activity diagram below shows the flow of execution when a user calls the DeleteSupplierCommand
together with the
details when this command is executed
Design Considerations
Due to the similarities of the design considerations between this command and the Add
command, please refer to the Design Considerations
section of the Add
command above for more information.
Find Command
In the following section, we will be going through how our find command works. Since the find command is also very
similar across all person types, we will be using the FindCustomerCommand
to illustrate how the find command
works. More information on the differences in finding Supplier
and Employee
can be found in our
User Guide
Current Implementation
Finding of customers can be divided into two steps: Parse
and Execute
.
Below is a partial class diagram for FindCustomerCommand
. Note that to make it more comprehensible, details not
related to FindCustomerCommand
are abstracted away.
The Sequence Diagram below illustrates the interactions within the Logic
component for the execute("findC Chetwin")
API call.
Parse
:
- When
Logic
is called upon to execute theFindCustomerCommand
, it uses theRhrhParser
class to parse the user command. - The
RhrhParser
then creates aFindCustomerCommandParser
to parse the command input. - If successful, the
FindCustomerCommandParser
then creates aCustomerClassContainsKeywordsPredicate
inModel
- This finally results in a
FindCustomerCommand
object being created from the newly createdCustomerClassContainsKeywordsPredicate
and is then executed by theLogicManager
.
Execute
:
- The instance of
FindCustomerCommand
created can communicate with theModel
when it is executed to filter theUniqueCustomerList
based on theCustomerClassContainsKeywordsPredicate
. - The result of the command execution is encapsulated as a
CommandResult
object which is returned back fromLogic
.
Flow of execution
The activity diagram below shows the flow of execution when a user calls the FindCustomerCommand
together with the
details when this command is executed
Design Considerations
Due to the similarities of the design considerations between this command and the Add
command, please refer to the Design Considerations
section of the Add
command above for more information.
Sort Command
In the following section, we will be going through how our sort command works. Since the sort command is also very
similar across all person types, we will be using the SortSupplierCommand
to illustrate how the sort command
works. More information on the differences in sorting Customer
and Employee
can be found in our
User Guide
Current Implementation
Sorting of a supplier list can be divided into two steps: Parse
and Execute
.
Here’s a partial class diagram for the SortSupplierCommand
. Note that to make it more comprehensible, details
not related to the SortSupplierCommand
are abstracted away.
The Sequence Diagram below illustrates the interactions within the Logic
component for the execute("sortS by/dd
o/a")
API call.
Parse
:
- When
Logic
is called upon to execute theSortSupplierCommand
, it uses theRhrhParser
class to parse the user command. - The
RhrhParser
then creates aSortSupplierCommandParser
to parse the command input. - If successful, this results in a
SortSupplierCommand
object being created which then is executed by theLogicManager
.
Execute
:
- The instance of
SortSupplierCommand
created can communicate with theModel
when it is executed to sort theUniqueSupplierList
based on a sorting type and sorting order. - The
Comparator
that is used to sort theUniqueSupplierList
is generated by theSupplierComparator
class - The result of the command execution is encapsulated as a
CommandResult
object which is returned back fromLogic
.
Flow of execution
The activity diagram below shows the flow of execution when a user calls the SortSupplierCommand
together with the
details when this command is executed
Design Considerations
Due to the similarities of the design considerations between this command and the Add
command, please refer to the Design Considerations
section of the Add
command above for more information.
Set Tables Command
Implementation
Set Tables command settables
takes in a list of numbers as arguments and creates a TableList that is stored in RHRH
A SetTablesCommand is created through the usage of our settables
command.
The process in which a new list of tables is set can be broken down into 2.
-
User input is sent into the RhrhParser and a SetTablesCommand is created.
-
The SetTablesCommand then proceeds to create and add the new tables to RHRH.
In the first step, the user input is parsed in SetTableCommandParser and broken down for other methods from other classes to create a SetTablesCommand. This is depicted in the Sequence Diagram below.
Once the SetTablesCommand is created, the LogicManager will then execute the SetTablesCommand and add the newly created list of tables to the model
Finally, a success message is printed to the user saying how many tables were set
Flow of execution:
The activity diagram below shows the flow of execution when a user calls this command together with the details when SetTablesCommand is executed
After user calls the command:
-
Command syntax and user input arguments are checked if they are valid
-
User input arguments are parsed and a SetTablesCommand is created and executed
During the SetTablesCommand execution:
-
The Table ID will be reset
-
All current reservations will be removed
-
New tables with the specified sizes will be created and added into a new list
-
New list of tables will overwrite the old list of tables in the TableManager inside the RHRH
Design Considerations
- User is allowed to format the argument for table sizes as a singular table size (e.g.
2
= 1 table that accommodates 2 people) or, with anx
for multiple tables with the same size (e.g.10x3
= 3 tables that accommodate 10 people)- This is because we find that in an actual restaurant, there will be many tables with the same sizes so this allows the user to set the tables faster without repeating the same table sizes many times. Hence, the design choice is to allow for these
x
syntax.
- This is because we find that in an actual restaurant, there will be many tables with the same sizes so this allows the user to set the tables faster without repeating the same table sizes many times. Hence, the design choice is to allow for these
- Table IDs will be reset to start from 1
- This is because we want the new set of tables to have the correct table IDs starting from 1 and not be affected by the old set of tables
- All current reservations will be removed
- This is because each reservation is linked to exactly one table so changing the tables in the restaurant will mess up the reservation and thus the reservations and tables will not be in sync. Hence, the design choice is to remove all reservations.
- There is no add/remove/edit table or table list
- Again, this is because reservations are linked to the tables themselves and if the user alters the list of tables, the reservations will not be in sync.
Alternatives considered:
Alternatively, we plan to allow editing of tables and allowing the user to shift reservations to specific tables if they want to overwrite the original assigned table.
Checks would have to be implemented to ensure that the reservations-table pairings are still valid.
This will allow for greater flexibility for restaurant managers where they can manually assign seats to certain reservations (e.g. Assigning a big table to a small group of VIPs even though the table space is not optimized)
Get corresponding customer command
For more information about this command and its usage, please refer to our User Guide
Implementation
Getting corresponding customer can be divided into two steps, Parse
and Execute
.
Parsing for this command is similar to any Delete Command, where we only need to parse for an INDEX
.
Hence, this section will focus on the executing aspect of this command.
The Sequence Diagram below illustrates how getC 1
is executed, assuming 1
is a valid reservation index.
- When a command is executed, it will interact with the
Model
to get the display reservation list. TheReservation
specified by the given index is obtained from the list. - A
CustomerContainsPhonePredicate
is instantiated with thePhone
of theReservation
, and it is used to update the filtered customer list to contain only the corresponding customer. - The result of the command execution is encapsulated as a
CommandResult
object and returned back to the caller. The Ui is also switched to customer view and display the corresponding customer.
Design Considerations
- Current design: We have a separate command to support viewing the information of a customer who made a reservation.
- Pros:
- There is no overlapping information between customer view and reservation view, hence less coupling between reservation and customer code, thus it improves coding quality.
- We can manipulate reservations and customers separately, which is more flexible.
- Cons:
- We need an extra step for viewing customer information corresponding to a reservation
- Pros:
- Alternative Design: Instead of a separate command, we can display the customer information with the reservation, or
display the reservations along with customer information.
- Pros:
- This implementation is more intuitive as we do not need an extra step to view corresponding customer information.
- Cons:
- It is harder to implement properly, as it will introduce more couplings to the codebase, espeacially when the database used is NoSQL, which hinders the ability to create relationship between entities.
- Pros:
[Proposed] Undo/redo feature
Proposed Implementation
The proposed undo/redo mechanism is facilitated by VersionedRhrh
. It extends Rhrh
with an undo/redo history, stored internally as an rhrhStateList
and currentStatePointer
. Additionally, it implements the following operations:
-
VersionedRhrh#commit()
— Saves the current RHRH state in its history. -
VersionedRhrh#undo()
— Restores the previous RHRH state from its history. -
VersionedRhrh#redo()
— Restores a previously undone RHRH state from its history.
These operations are exposed in the Model
interface as Model#commitRhrh()
, Model#undoRhrh()
and Model#redoRhrh()
respectively.
Given below is an example usage scenario and how the undo/redo mechanism behaves at each step.
Step 1. The user launches the application for the first time. The VersionedRhrh
will be initialized with the initial RHRH state, and the currentStatePointer
pointing to that single RHRH state.
Step 2. The user executes delete 5
command to delete the 5th person in RHRH. The delete
command calls Model#commitRhrh()
, causing the modified state of RHRH after the delete 5
command executes to be saved in the rhrhStateList
, and the currentStatePointer
is shifted to the newly inserted RHRH state.
Step 3. The user executes add n/David …
to add a new person. The add
command also calls Model#commitRhrh()
, causing another modified RHRH state to be saved into the rhrhStateList
.

Model#commitRhrh()
, so RHRH state will not be saved into the rhrhStateList
.
Step 4. The user now decides that adding the person was a mistake, and decides to undo that action by executing the undo
command. The undo
command will call Model#undoRhrh()
, which will shift the currentStatePointer
once to the left, pointing it to the previous RHRH state, and restores RHRH to that state.

currentStatePointer
is at index 0, pointing to the initial Rhrh state, then there are no previous Rhrh states to restore. The undo
command uses Model#canUndoRhrh()
to check if this is the case. If so, it will return an error to the user rather
than attempting to perform the undo.
The following sequence diagram shows how the undo operation works:

UndoCommand
should end at the destroy marker (X) but due to a limitation of PlantUML, the lifeline reaches the end of diagram.
The redo
command does the opposite — it calls Model#redoRhrh()
, which shifts the currentStatePointer
once to the right, pointing to the previously undone state, and restores RHRH to that state.

currentStatePointer
is at index rhrhStateList.size() - 1
, pointing to the latest RHRH state, then there are no undone Rhrh states to restore. The redo
command uses Model#canRedoRhrh()
to check if this is the case. If so, it will return an error to the user rather than attempting to perform the redo.
Step 5. The user then decides to execute the command list
. Commands that do not modify RHRH, such as list
, will usually not call Model#commitRhrh()
, Model#undoRhrh()
or Model#redoRhrh()
. Thus, the rhrhStateList
remains unchanged.
Step 6. The user executes clear
, which calls Model#commitRhrh()
. Since the currentStatePointer
is not pointing at the end of the rhrhStateList
, all RHRH states after the currentStatePointer
will be purged. Reason: It no longer makes sense to redo the add n/David …
command. This is the behavior that most modern desktop applications follow.
The following activity diagram summarizes what happens when a user executes a new command:
Design considerations:
Aspect: How undo & redo executes:
-
Alternative 1 (current choice): Saves the entire RHRH.
- Pros: Easy to implement.
- Cons: May have performance issues in terms of memory usage.
-
Alternative 2: Individual command knows how to undo/redo by
itself.
- Pros: Will use less memory (e.g. for
delete
, just save the person being deleted). - Cons: We must ensure that the implementation of each individual command are correct.
- Pros: Will use less memory (e.g. for
{more aspects and alternatives to be added}
Documentation, logging, testing, configuration, dev-ops
Appendix: Requirements
Product scope
Target user profile:
- managers of restaurant in charge of overseeing the list of customers, employees and suppliers
- has a need to manage a significant number of contacts
- prefer desktop apps over other types
- can type fast
- prefers typing to mouse interactions
- is reasonably comfortable using CLI apps
Value proposition: This application is created with the purpose of helping restaurant managers to manage the employees’ information (shift schedule, contact), diners (reservation timing, diner contacts) and suppliers (supplier meet up timing/weekly collection schedule, contacts), and at the same time manage contacts faster than a typical mouse/GUI driven app
User stories
Categories: Administrative, Customer, Employee, Supplier
Priorities: High, Low
As a … | I can … | so that … | Category | Priority |
---|---|---|---|---|
Manager | View specific table capacity | I can assign the correct group to the correct table | Administrative | High |
Manager | Update table availability | I can ensure that people will not assign any more customers to that table | Administrative | High |
Manager | Check table availability | I can assign a new group to the table | Administrative | High |
Manager | Add reservations (number of people, time, reserve table availability) | - | Customer | High |
Manager | Check if a particular timeslot is available for reservation | Organise the customer’s reservation slots | Customer | High |
Manager | Add/View/Change/Delete particulars for customer(number, initials, allergies, special requests, number of people etc.) | I can contact the customers and also provide them with personalised dining experiences | Customer | High |
Manager | View the amount of unclaimed loyalty points a customer has | I can give them the option of redeeming the points for a reward | Customer | High |
Manager | Change the amount of loyalty points a customer has | We can track the amount of loyalty points a customer has | Customer | High |
Manager | View reservations on a particular day | manage the reservations better | Customer | High |
Manager | Add customers to waiting list | Manage walk in customers | Customer | Low |
Manager | Add waiting list customers particulars | Organise walk in customers turn to enter the restaurant | Customer | Low |
Manager | View customer’s special payment method(vouchers) | I can keep track of finances correctly | Customer | Low |
Manager | Add/View/Change/Delete employees’ personal information | - | Employee | High |
Manager | View/Change the number of leaves remaining a particular employee has | I can ensure he is only claiming leaves that he is entitled to | Employee | High |
Manager | View/Change who is supposed to be on shift today | I know who is turning up for work today | Employee | High |
Manager | View/Change who is supposed to be on shift this week | I am aware of the week’s scheduling | Employee | High |
Manager | View/Change who is supposed to be on shift this month | I know who is turning up for work this month | Employee | High |
Manager | View work shifts that have yet to be filled | I can assign employees in to fill the manpower needs | Employee | High |
Manager | Add employees to available slots in a work shift | there would be no lack of personnel | Employee | High |
Manager | View/Change the monthly salary for a particular employee | I know if he is earning too much or too little | Employee | High |
Manager | View/Change the job type for a particular employee (full time, part time etc) | I know his payout scheme and if he deserves his pay | Employee | High |
Manager | View which shifts a particular employee is working in a week | I know when he should be turning up | Employee | Low |
Manager | Add observations of an employee during a shift | I can review them with him/her afterwards | Employee | Low |
Manager | View/Change number of hours worked by a particular employee per period | I can reward employees (employee of the month) | Employee | Low |
Manager | Add/View/Update/Delete supplier particular(name, contact info, food type) | - | Supplier | High |
Manager | View all suppliers delivering today | I can know who to expect today | Supplier | High |
Manager | View all suppliers delivering this week | I can know who to expect this week | Supplier | High |
Manager | View all deliveries for a particular supplier | I can know the deliveries made by the supplier | Supplier | High |
Manager | Add/View/Update/Delete delivery datetime by a particular supplier | I know what deliveries i will have for what time | Supplier | High |
Manager | View status of deliveries | I know which deliveries have been completed and which are outstanding | Supplier | High |
Manager | Update deliveries as done | I won’t accidentally wait for deliveries that have already been completed | Supplier | High |
Manager | View past deliveries | I can keep a receipt of the deliveries made in a specific period | Supplier | High |
Manager | View all suppliers by food type | I can know which supplier i have for a particular food type | Supplier | Low |
{More to be added}
Use cases
(For all use cases below, the System is the RHRH
and the Actor is the user
, unless specified otherwise)
Use case (UC01): Add a Customer
MSS
- User enters add customer command and keys in the customer’s details.
- Customer is added to RHRH.
-
System confirms that the customer has been added.
Use case ends.
Extensions
- 1a. System detects invalid command format.
- 1a1. System displays an error, showing unknown command.
- 1a2. User enters command again.
Steps 1a1 - 1a2 are repeated until the command is correctly formatted.
Use case resumes from step 2.
- 1b. System detects invalid command arguments
- 1b1. System displays error corresponding to the invalid argument and provides an example on the accepted format and arguments.
- 1b2. User enters command arguments again.
Steps 1b1 - 1b2 are repeated until all command arguments are valid.
Use case resumes from step 2.
- 2a. Customer to add already exists in RHRH.
- 2a1. System displays an error, and that the customer already exists in RHRH.
- 2a2. User enters customer details again.
Steps 2a1 - 2a2 are repeated until the customer to be added is unique.
Use case resumes from step 3.
Use case (UC02): Add an Employee
MSS
- User enters add employee command and keys in the employee’s details.
- Employee is added to RHRH.
-
System confirms that the employee has been added.
Use case ends.
Extensions
- 1a. System detects invalid command format.
- 1a1. System displays an error, showing unknown command.
- 1a2. User enters command again.
Steps 1a1 - 1a2 are repeated until the command is correctly formatted.
Use case resumes from step 2.
- 1b. System detects invalid command arguments.
- 1b1. System displays error corresponding to the invalid argument and provides an example on the accepted format and arguments.
- 1b2. User enters command arguments again.
Steps 1b1 - 1b2 are repeated until all command arguments are valid.
Use case resumes from step 2.
- 2a. Employee to add already exists in RHRH.
- 2a1. System displays an error, and that the employee already exists in RHRH.
- 2a2. User enters employee details again.
Steps 2a1 - 2a2 are repeated until the employee to be added is unique.
Use case resumes from step 3.
Use case (UC03): Add a Supplier
MSS
- User enters add supplier command and keys in the supplier’s details.
- Supplier is added to RHRH.
-
System confirms that the supplier has been added.
Use case ends.
Extensions
- 1a. System detects invalid command format.
- 1a1. System displays an error, showing unknown command.
- 1a2. User enters command again
Steps 1a1 - 1a2 are repeated until the command is correctly formatted.
Use case resumes from step 2.
- 1b. System detects invalid command arguments.
- 1b1. System displays error corresponding to the invalid argument and provides an example on the accepted format and arguments.
- 1b2. User enters command arguments again.
Steps 1b1 - 1b2 are repeated until all command arguments are valid.
Use case resumes from step 2.
- 2a. System detects that supplier to add already exists in RHRH.
- 2a1. System displays an error, and that the supplier already exists in RHRH.
- 2a2. User enters supplier details again.
Steps 2a1 - 2a2 are repeated until the supplier to be added is unique.
Use case resumes from step 3.
Use case (UC04): Add a reservation
MSS
- User enters add reservation command and keys in reservations details.
-
System confirms that reservation with the given details is added.
Use case ends
Extensions
- 1a. Tables are not set yet.
- 1a1. System requests user to set the tables.
- 1a2. User sets the tables (UC21)
Use case resumes from step 1.
- 1b. System detects invalid command format.
- 1b1. System displays an error, showing unknown command.
- 1b2. User enters command again.
Steps 1b1 - 1b2 are repeated until the command is correctly formatted.
Use case resumes from step 2.
- 1c. System detects invalid command arguments.
- 1c1. System displays error corresponding to the invalid argument and provides an example on the accepted format and arguments.
- 1c2. User enters command arguments again.
Steps 1c1 - 1c2 are repeated until the command arguments are valid.
Use case resumes from step 2.
- 1d. System is unable to find a reservation slot based on the date time entered.
- 1d1. System informs the user that the reservation slot is taken.
- 1d2. User enters the command again with another date time.
Steps 1d1 - 1d2 are repeated until there is a reservation slot available.
Use case resumes from step 2.
Use case (UC05): List all customers/employees/suppliers/reservations
MSS
- User enters the command to list all customers/employees/suppliers/reservations.
-
List of all customers/employees/suppliers/reservations is displayed in view.
Use case ends.
Extensions
- 1a. System detects invalid command format.
- 1a1. System displays an error, showing unknown command.
- 1a2. User enters command again.
Steps 1a1 - 1a2 are repeated until the command is correctly formatted.
Use case resumes from step 2.
Use case (UC06): Edit a Customer
MSS
- User enters the command to edit a customer and details of the customer to be edited.
- The specified customer is edited in RHRH.
-
System confirms that customer has been edited.
Use case ends.
Extensions
- 1a. System detects invalid command format.
- 1a1. System displays an error, showing unknown command.
- 1a2. User enters command again.
Steps 1a1 - 1a2 are repeated until the command is correctly formatted.
Use case resumes from step 2.
- 1b. System detects invalid command arguments.
- 1b1. System displays error corresponding to the invalid argument and provides an example on the accepted format and arguments.
- 1b2. User enters command arguments again.
Steps 1b1 - 1b2 are repeated until all command arguments are valid.
Use case resumes from step 2.
- 1c. System detects that customer to edit does not exist in RHRH.
- 1c1. System displays an error that no such customer index exist in RHRH.
- 1c2. User enters customer index again.
Steps 1c1 - 1c2 are repeated until the employee to be added is unique.
Use case resumes from step 2.
- 1d. System detects that editing of current customer would cause duplicate customers in RHRH.
- 1d1. System displays an error that customer already exists in RHRH.
- 1d2. User enters customer index again.
Steps 1d1 - 1d2 are repeated until the customer to be added is unique.
Use case resumes from step 2.
Use case (UC07): Edit an Employee
MSS
- User enters the command to edit an employee and details of the employee to be edited.
- The specified employee is edited in RHRH.
-
System confirms that employee has been edited.
Use case ends.
Extensions
- 1a. System detects invalid command format.
- 1a1. System displays an error, showing unknown command.
- 1a2. User enters command again.
Steps 1a1 - 1a2 are repeated until the command is correctly formatted.
Use case resumes from step 2.
- 1b. System detects invalid command arguments.
- 1b1. System displays error corresponding to the invalid argument and provides an example on the accepted format and arguments
- 1b2. User enters command arguments again.
Steps 1b1 - 1b2 are repeated until all command arguments are valid.
Use case resumes from step 2.
- 1c. System detects that employee to edit does not exist in RHRH.
- 1c1. System displays an error that no such employee index exist in RHRH.
- 1c2. User enters employee index again.
Steps 1c1 - 1c2 are repeated until the employee to be added is unique.
Use case resumes from step 2.
- 1d. System detects that editing of current employee would cause duplicate employees in RHRH.
- 1d1. System displays an error that employee already exists in RHRH.
- 1d2. User enters employee index again.
Steps 1d1 - 1d2 are repeated until the employee to be added is unique.
Use case resumes from step 2.
Use case (UC08): Edit a Supplier
MSS
- User enters the command to edit a supplier and details of the supplier to be edited.
- The specified supplier is edited in RHRH.
-
System confirms that supplier has been edited.
Use case ends.
Extensions
- 1a. System detects invalid command format.
- 1a1. System displays an error, showing unknown command.
- 1a2. User enters command again.
Steps 1a1 - 1a2 are repeated until the command is correctly formatted.
Use case resumes from step 2.
- 1b. System detects invalid command arguments.
- 1b1. System displays error corresponding to the invalid argument and provides an example on the accepted format and arguments.
- 1b2. User enters command arguments again.
Steps 1b1 - 1b2 are repeated until all command arguments are valid.
Use case resumes from step 2.
- 1c. System detects that supplier to edit does not exist in RHRH.
- 1c1. System displays an error that no such supplier index exist in RHRH.
- 1c2. User enters supplier index again.
Steps 1c1 - 1c2 are repeated until the supplier to be added is unique.
Use case resumes from step 2.
- 1d. System detects that editing of current supplier would cause duplicate suppliers in RHRH.
- 1d1. System displays an error that supplier already exists in RHRH.
- 1d2. User enters supplier index again.
Steps 1d1 - 1d2 are repeated until the supplier to be added is unique.
Use case resumes from step 2.
Use case (UC09): Edit a reservation
MSS
- User enters the command to edit reservation fields.
- The specified reservation is edited in RHRH.
-
System confirms that the reservation has been edited and shows the edited reservation.
Use case ends
Extensions
- 1a. System detects invalid command format.
- 1a1. System displays an error, showing unknown command.
- 1a2. User enters command again.
Steps 1a1 - 1a2 are repeated until the command is correctly formatted.
Use case resumes from step 2.
- 1b. System detects invalid command arguments.
- 1b1. System displays error corresponding to the invalid argument and provides an example on the accepted format and arguments.
- 1b2. User enters command arguments again.
Steps 1b1 - 1b2 are repeated until all command arguments are valid.
Use case resumes from step 2.
- 1c. System detects that reservation to edit does not exist in RHRH.
- 1c1. System displays an error that no such reservation index exist in RHRH.
- 1c2. User enters reservation index again.
Steps 1c1 - 1c2 are repeated until the reservation to be added is unique.
Use case resumes from step 2.
Use case (UC10): Delete a Customer
MSS
- User enters the command to delete a customer.
- The specified customer is deleted from RHRH.
-
System confirms that the customer has been deleted.
Use case ends.
Extensions
- 1a. System detects invalid command format.
- 1a1. System displays an error, showing unknown command.
- 1a2. User enters command again.
Steps 1a1 - 1a2 are repeated until the command is correctly formatted.
Use case resumes from step 2.
- 1b. System detects invalid customer index.
- 1b1. System displays error corresponding to the invalid index and provides an example on the accepted format and arguments.
- 1b2. User enters customer index again.
Steps 1b1 - 1b2 are repeated until all command arguments are valid.
Use case resumes from step 2.
- 1c. System detects that customer to delete does not exist in RHRH.
- 1c1. System displays an error that no such customer index exist in RHRH.
- 1c2. User enters customer index again
Steps 1c1 - 1c2 are repeated until the customer to delete exists in RHRH.
Use case resumes from step 2.
Use case (UC11): Delete an Employee
MSS
- User enters the command to delete an employee.
- The specified employee is deleted from RHRH.
-
System confirms that the employee has been deleted.
Use case ends.
Extensions
- 1a. System detects invalid command format.
- 1a1. System displays an error, showing unknown command.
- 1a2. User enters command again.
Steps 1a1 - 1a2 are repeated until the command is correctly formatted.
Use case resumes from step 2.
- 1b. System detects invalid employee index.
- 1b1. System displays error corresponding to the invalid index and provides an example on the accepted format and arguments.
- 1b2. User enters employee index again.
Steps 1b1 - 1b2 are repeated until all command arguments are valid.
Use case resumes from step 2.
- 1c. System detects that employee to delete does not exist in RHRH.
- 1c1. System displays an error that no such employee index exist in RHRH.
- 1c2. User enters employee index again
Steps 1c1 - 1c2 are repeated until the employee to delete exists in RHRH.
Use case resumes from step 2.
Use case (UC12): Delete a Supplier
MSS
- User enters the command to delete a supplier.
- The specified supplier is deleted from RHRH.
-
System confirms that the supplier has been deleted.
Use case ends.
Extensions
- 1a. System detects invalid command format.
- 1a1. System displays an error, showing unknown command.
- 1a2. User enters command again.
Steps 1a1 - 1a2 are repeated until the command is correctly formatted.
Use case resumes from step 2.
- 1b. System detects invalid supplier index.
- 1b1. System displays error corresponding to the invalid index and provides an example on the accepted format and arguments.
- 1b2. User enters supplier index again.
Steps 1b1 - 1b2 are repeated until all command arguments are valid.
Use case resumes from step 2.
- 1c. System detects that supplier to delete does not exist in RHRH.
- 1c1. System displays an error that no such supplier index exist in RHRH.
- 1c2. User enters supplier index again
Steps 1c1 - 1c2 are repeated until the supplier to delete exists in RHRH.
Use case resumes from step 2.
Use case (UC13): Delete a Reservation
MSS
- User enters the command to delete a reservation.
- The specified reservation is deleted from RHRH.
-
System confirms that the reservation has been deleted.
Use case ends.
Extensions
- 1a. System detects invalid command format.
- 1a1. System displays an error, showing unknown command.
- 1a2. User enters command again.
Steps 1a1 - 1a2 are repeated until the command is correctly formatted.
Use case resumes from step 2.
- 1b. System detects invalid reservation index.
- 1b1. System displays error corresponding to the invalid index and provides an example on the accepted format and arguments.
- 1b2. User enters reservation index again.
Steps 1b1 - 1b2 are repeated until all command arguments are valid.
Use case resumes from step 2.
- 1c. System detects that reservation to delete does not exist in RHRH.
- 1c1. System displays an error that no such reservation index exist in RHRH.
- 1c2. User enters reservation index again
Steps 1c1 - 1c2 are repeated until the reservation to delete exists in RHRH.
Use case resumes from step 2.
Use case (UC14): Find Customers in RHRH
MSS
- User enters the command to find the customers in RHRH based on a sequence of keywords.
- The customer list is filtered in RHRH based on matching of keywords.
-
System confirms that the customer list has been filtered and displays number of filtered customers.
Use case ends.
Extensions
- 1a. System detects invalid command format.
- 1a1. System displays an error, showing unknown command.
- 1a2. User enters command again.
Steps 1a1 - 1a2 are repeated until the command is correctly formatted.
Use case resumes from step 2.
- 1b. System detects empty or invalid keywords.
- 1b1. System displays error corresponding to the empty or invalid keywords and provides an example on the accepted format and arguments.
- 1b2. User enters command arguments again.
Steps 1b1 - 1b2 are repeated until all command arguments are valid.
Use case resumes from step 2.
Use case (UC15): Find Employees in RHRH
MSS
- User enters the command to find the employees in RHRH based on a sequence of keywords.
- The employee list is filtered in RHRH based on matching of keywords.
-
System confirms that the employee list has been filtered and displays number of filtered employees.
Use case ends.
Extensions
- 1a. System detects invalid command format.
- 1a1. System displays an error, showing unknown command.
- 1a2. User enters command again.
Steps 1a1 - 1a2 are repeated until the command is correctly formatted.
Use case resumes from step 2.
- 1b. System detects empty or invalid keywords.
- 1b1. System displays error corresponding to the empty or invalid keywords and provides an example on the accepted format and arguments.
- 1b2. User enters command arguments again.
Steps 1b1 - 1b2 are repeated until all command arguments are valid.
Use case resumes from step 2.
Use case (UC16): Find Suppliers in RHRH
- User enters the command to find the suppliers in RHRH based on a sequence of keywords.
- The supplier list is filtered in RHRH based on matching of keywords.
-
System confirms that the supplier list has been filtered and displays number of filtered suppliers.
Use case ends.
Extensions
- 1a. System detects invalid command format.
- 1a1. System displays an error, showing unknown command.
- 1a2. User enters command again.
Steps 1a1 - 1a2 are repeated until the command is correctly formatted.
Use case resumes from step 2.
- 1b. System detects empty or invalid keywords.
- 1b1. System displays error corresponding to the empty or invalid keywords and provides an example on the accepted format and arguments.
- 1b2. User enters command arguments again.
Steps 1b1 - 1b2 are repeated until all command arguments are valid.
Use case resumes from step 2.
Use case (UC17): Check for reservations made for a date and time
MSS
- User enters the command to check the reservations made for a date and time.
-
System shows the reservations that are on that date and time.
Use case ends.
Extensions:
- 1a. System detects invalid command format of date/time.
- 1a1. System displays an error, showing unknown command.
- 1a2. User enters command again.
Steps 1a1 - 1a2 are repeated until the command is correctly formatted.
Use case resumes from step 2.
- 1b. System detects that there are no reservations made for that date and time
-
1b1. No reservations are displayed
Use case ends
-
Use case (UC18): Check for reservations made for a date
MSS
- User enters the command to check the reservations made for a date.
-
System shows the reservations that are on that date.
Use case ends
Extensions:
- 1a. System detects invalid command format of date/time.
- 1a1. System displays an error, showing unknown command.
- 1a2. User enters command again.
Steps 1a1 - 1a2 are repeated until the command is correctly formatted.
Use case resumes from step 2.
- 1b. System detects that there are no reservations made for that date
-
1b1. No reservations are displayed
Use case ends
-
Use case (UC19): Check for reservations made for a time on the current date
MSS
- User enters the command to check the reservations made for a time on the current date.
-
System shows the reservations that are on that time on the current date
Use case ends
Extensions:
- 1a. System detects invalid command format of date/time.
- 1a1. System displays an error, showing unknown command.
- 1a2. User enters command again.
Steps 1a1 - 1a2 are repeated until the command is correctly formatted.
Use case resumes from step 2.
- 1b. System detects that there are no reservations made for that time on the current date
-
1b1. No reservations are displayed
Use case ends
-
Use case (UC20): Get customer who made a reservation in RHRH
MSS
- User enters the command to get the customer who made a specified reservation.
- The corresponding customer information is shown.
-
System confirms that the corresponding customer is listed.
Use case ends.
Extension
- 1a. System detects invalid command format.
- 1a1. System displays an error, showing unknown command.
- 1a2. User enters command again.
Steps 1a1 - 1a2 are repeated until the command is correctly formatted.
Use case resumes from step 2.
- 1b. System detects invalid reservation index.
- 1b1. System displays error corresponding to the invalid index and provides an example on the accepted format and arguments.
- 1b2. User enters reservation index again.
Steps 1b1 - 1b2 are repeated until all command arguments are valid.
Use case resumes from step 2.
- 1c. System detects that reservation to get customer information does not exist in RHRH.
- 1c1. System displays an error that no such reservation index exist in RHRH.
- 1c2. User enters reservation index again
Steps 1c1 - 1c2 are repeated until the reservation to get customer information exists in RHRH.
Use case resumes from step 2.
Use case (UC21): Set the tables of the restaurant inside the app
MSS
- User enters the command to set the tables in RHRH.
-
System shows the number of tables set in the restaurant.
Use case ends
Extensions:
- 1a. System detects invalid command format.
- 1a1. System displays an error, showing unknown command.
- 1a2. User enters command again.
Steps 1a1 - 1a2 are repeated until the command is correctly formatted.
Use case resumes from step 2.
Use case (UC22): Sort Customers in RHRH
MSS
- User enters the command to sort the customer list in RHRH based on a sorting type and order.
- The customer list is sorted in RHRH according to specified sorting type and order.
-
System confirms that the customers have been sorted.
Use case ends.
Extensions
- 1a. System detects invalid command format.
- 1a1. System displays an error, showing unknown command.
- 1a2. User enters command again.
Steps 1a1 - 1a2 are repeated until the command is correctly formatted.
Use case resumes from step 2.
- 1b. System detects invalid customer sorting type and order.
- 1b1. System displays error corresponding to the invalid argument and provides an example on the accepted format and arguments.
- 1b2. User enters command arguments again.
Steps 1b1 - 1b2 are repeated until all command arguments are valid.
Use case resumes from step 2.
- 1c. System detects that there are no customers to sort in the current list.
-
1c1. System displays an error that there are no customers to sort in the current list.
Use case ends.
-
Use case (UC23): Sort Employees in RHRH
MSS
- User enters the command to sort the employee list in RHRH based on a sorting type and order.
- The employee list is sorted in RHRH according to specified sorting type and order.
-
System confirms that the employees have been sorted.
Use case ends.
Extensions
- 1a. System detects invalid command format.
- 1a1. System displays an error, showing unknown command.
- 1a2. User enters command again.
Steps 1a1 - 1a2 are repeated until the command is correctly formatted.
Use case resumes from step 2.
- 1b. System detects invalid employee sorting type and order.
- 1b1. System displays error corresponding to the invalid argument and provides an example on the accepted format and arguments.
- 1b2. User enters command arguments again.
Steps 1b1 - 1b2 are repeated until all command arguments are valid.
Use case resumes from step 2.
- 1c. System detects that there are no employees to sort in the current list.
-
1c1. System displays an error that there are no employees to sort in the current list.
Use case ends.
-
Use case (UC24): Sort Suppliers in RHRH
MSS
- User enters the command to sort the supplier list in RHRH based on a sorting type and order.
- The supplier list is sorted in RHRH according to specified sorting type and order.
-
System confirms that the suppliers have been sorted.
Use case ends.
Extensions
- 1a. System detects invalid command format.
- 1a1. System displays an error, showing unknown command.
- 1a2. User enters command again.
Steps 1a1 - 1a2 are repeated until the command is correctly formatted.
Use case resumes from step 2.
- 1b. System detects invalid supplier sorting type and order.
- 1b1. System displays error corresponding to the invalid argument and provides an example on the accepted format and arguments.
- 1b2. User enters command arguments again.
Steps 1b1 - 1b2 are repeated until all command arguments are valid.
Use case resumes from step 2.
- 1c. System detects that there are no suppliers to sort in the current list.
-
1c1. System displays an error that there are no suppliers to sort in the current list.
Use case ends.
-
Use case (UC25): Reset all customers/employees/suppliers in default sorting state
MSS
- User enters the command to reset all customers/employees/suppliers in default sorting state.
-
List of all customers/employees/suppliers in default sorting state is displayed in view.
Use case ends.
Extensions
- 1a. System detects invalid command format.
- 1a1. System displays an error, showing unknown command.
- 1a2. User enters command again.
Steps 1a1 - 1a2 are repeated until the command is correctly formatted.
Use case resumes from step 2.
Non-Functional Requirements
- Should work on any mainstream OS as long as it has Java
11
or above installed. - Should be able to hold up to 1000 persons without a noticeable sluggishness in performance for typical usage.
- A user with above average typing speed for regular English text (i.e. not code, not system admin commands) should be able to accomplish most of the tasks faster using commands than using the mouse.
- Should not be stored in a Database Management System (DBMS).
- Should be stored locally and can be manually edited by the user.
- Program should be delivered to users in a single JAR file.
- Program should be able to run with just a double-click and no CLI required.
- Should be runnable on both
32-bit
and64-bit
processors. - New users should be able to navigate and use the program effectively.
- Software should not exceed 100MB.
- Documents should not exceed 15MB per file.
- Developer Guide and User Guide should be PDF-friendly(no expandable panels, embedded videos, animated GIFs etc.).
- Does not require a server component as the software will not perform automated tasks.
Glossary
- Address : Address of the contact
- Allergies : Any allergies of the customer
- Contact : A contact containing details about the particular person
- Customer : One of the types of contact, used to represent a customer of the restaurant
- Delivery Details : The delivery dates/times of a supplier
- Leaves : Leaves of the employee
- Loyalty points : Points stored by each customer
- Email : Email of the contact
- Employee : One of the types of contact, used to represent an employee of the restaurant
- Job title : The different job titles of employees (e.g. Part-time, Full-time etc.)
- Mainstream OS : Windows, Linux, Unix, OS-X
- Name : Name of the contact
- Phone : Phone number of the contact
- Salary : Salary of an employee
- Shift : Time slot that employee is supposed to be working
- Special Request : Additional information of the customer
- Supplier : One of the types of contact, used to represent a supplier of the restaurant
- Supply Type : The type of food supply a supplier provides
- Remark : Additional information of a reservation
- Table : Contains a size and a table ID, and is used to ensure that each reservation has an available table
- Tag : Additional information to label a contact with
Appendix: Instructions for manual testing
Given below are instructions to test the app manually.

Launch and shutdown
-
Initial launch
-
Download the jar file and copy into an empty folder
-
Double-click the jar file
Expected: Shows the GUI with a set of sample contacts. The window size may not be optimum.
-
-
Saving window preferences
-
Resize the window to an optimum size. Move the window to a different location. Close the window.
-
Re-launch the app by double-clicking the jar file.
Expected: The most recent window size and location is retained.
-
-
Exiting the app
- While the app is still running, enter the
exit
command.
Expected: The app closes.
- While the app is still running, enter the
Adding a customer/employee/supplier
-
Add a Customer to the Customer list
- Test case:
addC n/John Doe p/87654321 e/e12345@u.nus.edu a/30 Geylang Drive lp/1000 alg/Kiwi sr/outdoors
Expected: Customer John Doe has been added to the Customer list. Details of the Customer John Doe will be shown on the result display area. - Test case:
addC n/John Doey
Expected: Customer will not be added, andInvalid command format! ...
where...
is the correct command format. Error is thrown due to missing compulsory fields. - Test case:
addC n/J@hn p/87654321 e/e12345@u.nus.edu a/30 Geylang Drive lp/1000 alg/Kiwi sr/outdoors
Expected: Customer will not be added, and an error messageNames should only contain alphanumeric characters and spaces, and it should not be blank
will be shown due to @ being non-alphanumeric. - Test case:
addC n/John Doe p/87a2ff e/e12345@u.nus.edu a/30 Geylang Drive lp/1000 alg/Kiwi sr/outdoors
Expected: Customer will not be added, and an error messagePhone numbers should only contain numbers, and it should be at least 3 digits long
will be shown due to alphabets being in the phone number field. - Test case:
addC n/John Doe p/87654321 e/e12345.LOL a/30 Geylang Drive lp/1000 alg/Kiwi sr/outdoors
Expected: Customer will not be added, and an error message that tells the user the correct email format will be displayed. - Test case:
addC n/John Doe p/87654321 e/e12345@u.nus.edu a/ lp/1000 alg/Kiwi sr/outdoors
Expected: Customer will not be added, and an error messageAddresses can take any values, and it should not be blank
will be shown due to address being left blank. - Test case:
addC n/John Doe p/87654321 e/e12345@u.nus.edu a/30 Geylang Drive lp/-2 alg/Kiwi sr/outdoors
Expected: Customer will not be added, and an error messageLoyalty points must be numerical, more than or equals to 0 and less than or equals to 100000
will be displayed due to loyalty points being negative. - Test case:
addC n/John Doe p/87654321 e/e12345@u.nus.edu a/30 Geylang Drive lp/1000 alg/*Kiwi* sr/outdoors
Expected: Customer will not be added, and an error messageAllergies names should be alphanumeric
will be displayed due to * being non-alphanumeric. - Test case:
addC n/John Doe p/87654321 e/e12345@u.nus.edu a/30 Geylang Drive lp/1000 alg/Kiwi sr/**outdoors**
Expected: Customer will not be added, and an error messageSpecialRequests names should be alphanumeric
will be displayed due to * being non-alphanumeric.
- Test case:
-
Add an Employee to the Employee List
- Test case:
addE n/John Doe p/87654321 e/e12345@u.nus.edu a/30 Geylang Drive sal/4000 jt/Head Chef l/14 sh/2021-12-08 0800
Expected: Employee John Doe has been added to the Employee List. Details of Employee John Doe will be shown on the result display area. - Test case:
addE n/John Doey
Expected: Employee will not be added, andInvalid command format! ...
where...
is the correct command format. Error is thrown due to missing compulsory fields. - Test case:
addE n/John Doe p/87654321 e/e12345@u.nus.edu a/30 Geylang Drive sal/4000 jt/#Head Chef l/14 sh/2021-12-08 0800
Expected: Employee will not be added, and an error messagejob titles should only contain alphanumeric characters and spaces, and should not be left blank
will be shown due to # being non-alphanumeric. - Test case:
addE n/John Doe p/87654321 e/e12345@u.nus.edu a/30 Geylang Drive sal/-100 jt/Head Chef l/14 sh/2021-12-08 0800
Expected: Employee will not be added, and an error messageSalary should be numerical and more than or equal to 100 and less than or equals to 10 million
will be shown due to the salary being negative. - Test case:
addE n/John Doe p/87654321 e/e12345@u.nus.edu a/30 Geylang Drive sal/4000 jt/Head Chef l/700 sh/2021-12-08 0800
Expected: Employee will not be added, and an error messageLeaves should be numerical and be more than or equals to 0, less than or equals to 365
will be shown due to the number of leaves entitled exceeding 1 year. Test case:addE n/John Doe p/87654321 e/e12345@u.nus.edu a/30 Geylang Drive sal/4000 jt/Head Chef l/14 sh/2021-12-08 2pm
Expected: Employee will not be added, and an error message that shows the user the correct format for entering shifts will be displayed, due to the error in formatting for time.
- Test case:
-
Add a Supplier to the Supplier List
- Test case:
addS n/John Doe p/87654321 e/e12345@u.nus.edu a/30 Geylang Drive dd/2021-12-08 1200 st/Beef
Expected: Supplier John Doe has been added to the Supplier List. Details of the Supplier John Doe will be shown on the result display area. - Test case:
addS n/John Doey
Expected: Supplier will not be added, andInvalid command format! ...
where...
is the correct command format. Error is thrown due to missing compulsory fields. - Test case:
addS n/John Doe p/87654321 e/e12345@u.nus.edu a/30 Geylang Drive dd/2021-12-40 1200 st/Beef
Expected: Supplier will not be added, and an error message that shows the user the correct format for entering delivery details will be displayed, due to the error caused by entering an invalid day. - Test case:
addS n/John Doe p/87654321 e/e12345@u.nus.edu a/30 Geylang Drive dd/2021-14-12 1200 st/Beef
Expected: Supplier will not be added, and an error message that shows the user the correct format for entering delivery details will be displayed, due to the error caused by entering an invalid month. - Test case:
addS n/John Doe p/87654321 e/e12345@u.nus.edu a/30 Geylang Drive dd/2021-12-08 1200 st/#@$Beef
Expected: Supplier will not be added, and an error messageSupply types should only contain alphanumeric characters and spaces, and it should not be blank
will be shown due to the presence of non-alphanumerical characters in the supply type.
- Test case:
Adding a reservation
-
The tables are already set and there are still available tables.
- Prerequisites: The tables are already set using
setTables
. Please refer to our User Guide for the command usage. - Test case:
addr 2 p/PHONE at/24-12-2020 2000 r/some remark t/some tag t/another tag
, wherePHONE
must belong to a customer in the customer list, and there is an available table for 2 on24-12-2020 2000
Expected: a new reservation with the specified description is added to the reservation list. - Test case:
addr 3 p/PHONE at/24-12-2020 1900
, wherePHONE
must belong to a customer in the customer list, and there is an available table for 3 on24-12-2020 1900
Expected: a new reservation with the specified description is added to the reservation list. - Test case:
addr 5 p/PHONE at/DATE_TIME
, wherePHONE
andDATE_TIME
belong one of the following case:-
PHONE
does not belong to any customer in the reservation list,DATE_TIME
is valid (of correct format and there is an available table for 5 at that time) -
PHONE
is valid (belong to a customer) butDATE_TIME
is invalid (of incorrect format or the restaurant is full at that time)
Expected: No reservation is added. Error details shown in the status message. Status bar remains the same.
-
- Prerequisites: The tables are already set using
-
The tables are not set yet.
- Prerequisite: the
tables
array in the data file is empty. - Repeat the same test cases as 1.2 and 1.3.
Expected: No reservation is added. Error message shown, telling users to set tables before use.
- Prerequisite: the
Listing customers/employees/suppliers/reservations
-
Switching the view to display the customer/employee/supplier/reservation list.
- Test case:
listC
/listE
/listS
/listR
Expected: List of all customers/employees/suppliers/reservations will be displayed. Success messageSwitched to X View
will be displayed (where X refers to eitherCustomer
,Employee
,Supplier
orReservation
depending on whichlist
command is used). - Test case:
lisC
/leestE
/ …
Expected: Any form of misspelling will result in no changes to the current view. Error messageUnknown command
will be displayed.
- Test case:
Deleting a customer/employee/supplier/reservation
-
Deleting a customer/employee/supplier/reservation while all customers/employees/suppliers/reservation are being shown.
- Prerequisites: List all customers/employees/suppliers/reservations using the
listC
/listE
/listS
/listR
command. - Test case:
deleteC 1
/deleteE 1
/deleteS 1
/deleteR 1
Expected: First customer/employee/supplier/reservation is deleted from the list. Details of the deleted customer/employee/supplier/reservation shown in the results display. - Test case:
deleteC 0
/deleteE -1
/deleteS -50
/deleteR 0
Expected: Nobody is deleted. Error details shown in the results display with a result messageInvalid comamnd format...
- Other incorrect delete commands to try:
deleteS
,deleteC x
,delete 1
(where x is an integer larger than the customer list size)
Expected: Nobody is deleted. Error details shown in the results display. EnteringdeleteS
will produce the same error message as test case 1.3 while enteringdeleteC x
(where x is an integer larger than the customer list size) anddelete 1
will produce the error messageCustomer index is invalid
andUnknown command
respectively.
- Prerequisites: List all customers/employees/suppliers/reservations using the
-
Deleting a customer/employee/supplier/reservation while customer list is filtered.
- Prerequisites: Filter display list by using the
findC KEYWORD
/findE KEYWORD
/findS KEYWORD
/check DATE
command, whereKEYWORD
must exist in at least 1 of the customers/employees/suppliers and there must be at least 1 reservation onDATE
. - Repeat the same test cases as 1.2 to 1.4.
- Prerequisites: Filter display list by using the
Editing a customer
-
Editing a customer while all customers are being shown.
- Prerequisites: List all customers using the
listC
command. - Test case:
editC 1 n/Chetwin lp/5000
Expected: First customer in the list will be updated to have “Chetwin” and “5000” as hisname
andloyalty points
respectively. Details of the edited customer shown in the results display. - Test case:
editC -1 e/chetwinlow@gmail.com
Expected: Nobody is edited. Error details shown in the results display with a result messageInvalid comamnd format...
- Test case:
editC 1
Expected: Nobody is edited. Error details shown in the results display with a result messageAt least one field to edit must be provided.
- Other incorrect edit commands to try:
editC
/editC f/invalid prefix
/edit 1
/editC x n/john
(where x is larger than the customer list size)
Expected: Nobody is edited. Error details shown in the results display. Entering the first 2 commands will produce the same error message as test case 1.3 while enteringedit 1
will produce the error messageUnknown command
and the last one resulting in error messageThe customer index provided is invalid
- Prerequisites: List all customers using the
-
Editing a customer while customer list is filtered.
- Prerequisites: Filter display list by using the
findC KEYWORD
command, whereKEYWORD
must exist in at least 1 of the customers. - Repeat the same test cases as 1.2 to 1.4.
- Prerequisites: Filter display list by using the
Editing a supplier
-
Editing a supplier while all suppliers are being shown.
- Prerequisites: List all suppliers using the
listS
command. - Test case:
editS 1 n/Chetwin st/chicken
Expected: First customer in the list will be updated to have “Chetwin” and “chicken” as hisname
andsupply type
respectively. Details of the edited supplier shown in the results display. - Test case:
editS -1 e/chetwinlow@gmail.com
Expected: Nobody is edited. Error details shown in the results display with a result messageInvalid comamnd format...
- Test case:
editS 1
Expected: Nobody is edited. Error details shown in the results display with a result messageAt least one field to edit must be provided.
- Other incorrect edit commands to try:
editS
/editS f/invalid prefix
/edit 1
/editS x n/john
(where x is larger than the supplier list size)
Expected: Nobody is edited. Error details shown in the results display. Entering the first 2 commands will produce the same error message as test case 1.3 while enteringedit 1
will produce the error messageUnknown command
and the last one resulting in error messageThe supplier index provided is invalid
- Prerequisites: List all suppliers using the
-
Editing a supplier while supplier list is filtered.
- Prerequisites: Filter display list by using the
findS KEYWORD
command, whereKEYWORD
must exist in at least 1 of the suppliers. - Repeat the same test cases as 1.2 to 1.4.
- Prerequisites: Filter display list by using the
Editing an employee
-
Editing an employee while all employees are being shown.
- Prerequisites: List all employees using the
listE
command. - Test case:
editE 1 n/Chetwin s/5000
Expected: First employee in the list will be updated to have “Chetwin” and “5000” as hisname
andsalary
respectively. Details of the edited employee shown in the results display. - Test case:
editE -1 e/chetwinlow@gmail.com
Expected: Nobody is edited. Error details shown in the results display with a result messageInvalid comamnd format...
- Test case:
editE 1
Expected: Nobody is edited. Error details shown in the results display with a result messageAt least one field to edit must be provided.
- Other incorrect edit commands to try:
editE
/editE f/invalid prefix
/edit 1
/editE x n/john
(where x is larger than the employee list size)
Expected: Nobody is edited. Error details shown in the results display. Entering the first 2 commands will produce the same error message as test case 1.3 while enteringedit 1
will produce the error messageUnknown command
and the last one resulting in error messageThe employee index provided is invalid
- Prerequisites: List all employees using the
-
Editing an employee while employee list is filtered.
- Prerequisites: Filter display list by using the
findE KEYWORD
command, whereKEYWORD
must exist in at least 1 of the employees. - Repeat the same test cases as 1.2 to 1.4.
- Prerequisites: Filter display list by using the
Finding customers
-
Finding the customers
- Test case:
findC Chetwin
Expected: The customer list is filtered to only show customers that match “Chetwin”(case-insensitive) in any of its fields. Success message shown in results display. - Test case:
findC Chetwin 15
Expected: The customer list is filtered to only show customers that match “Chetwin”(case-insensitive) and “15” in any of its fields cumulatively. Success message shown in results display. - Test case:
findC Chetwin 15 913045
Expected: The customer list is filtered to only show customers that match “Chetwin”(case-insensitive) and “15” and “913045” in any of its fields cumulatively. Success message shown in results display. - Test case:
findC
Expected: Customer list is not filtered. Error details shown in the result display, with a result messageInvalid command format
- Test case:
Finding suppliers
-
Finding the suppliers
- Test case:
findS Chetwin
Expected: The supplier list is filtered to only show customers that match “Chetwin”(case-insensitive) in any of its fields. Success message shown in results display. - Test case:
findS Chetwin 15
Expected: The list is filtered to only show suppliers that match “Chetwin”(case-insensitive) and “15” in any of its fields cumulatively. Success message shown in results display. - Test case:
findS Chetwin 15 913045
Expected: The supplier list is filtered to only show customers that match “Chetwin”(case-insensitive) and “15” and “913045” in any of its fields cumulatively. Success message shown in results display. - Test case:
findS
Expected: Supplier list is not filtered. Error details shown in the result display, with a result messageInvalid command format
- Test case:
Finding employees
-
Finding the employees
- Test case:
findE Chetwin
Expected: The employee list is filtered to only show customers that match “Chetwin”(case-insensitive) in any of its fields. Success message shown in results display. - Test case:
findE Chetwin 15
Expected: The employee list is filtered to only show suppliers that match “Chetwin 15”(case-insensitive) in any of its fields. Success message shown in results display. - Test case:
findE Chetwin 15 913045
Expected: The employee list is filtered to only show customers that match “Chetwin 15 913045” in any of its fields. Success message shown in results display. - Test case:
findE
Expected: Supplier list is not filtered. Error details shown in the result display, with a result messageInvalid command format
- Test case:
Get the corresponding customer of a reservation
-
Getting the corresponding customer of a reservation while all reservations are shown
- Prerequisites: List all reservations using
listR
- Test case:
getC 1
Expected: The customer who made the first reservation is displayed. - Test case:
getC 0
Expected: No customer is shown. Error details are shown in the status message. Status bar remains the same. - Other incorrect commands to try:
getC
,getC -1
,getC x
(where x is larger than the customer list size),...
Expected: Similar to previous.
- Prerequisites: List all reservations using
-
Getting the corresponding customer of a reservation while reservation list is filtered.
- Prerequisites: Filter display reservation list using
check DATE
, where there must be at least 1 reservation onDATE
. - Repeat the same test cases as 1.2 to 1.4.
- Prerequisites: Filter display reservation list using
Get the corresponding customer of a reservation
-
Getting the corresponding customer of a reservation while all reservations are shown
- Prerequisites: List all reservations using
listR
- Test case:
getC 1
Expected: The customer who made the first reservation is displayed. - Test case:
getC 0
Expected: No customer is shown. Error details are shown in the status message. Status bar remains the same. - Other incorrect commands to try:
getC
,getC -1
,getC x
(where x is larger than the customer list size),...
Expected: Similar to previous.
- Prerequisites: List all reservations using
-
Getting the corresponding customer of a reservation while reservation list is filtered.
- Prerequisites: Filter display reservation list using
check DATE
, where there must be at least 1 reservation onDATE
. - Repeat the same test cases as 1.2 to 1.4.
- Prerequisites: Filter display reservation list using
Setting the tables
-
Test case:
settables 10x4,2,2,1
Expected: Result display shows 7 tables are set and all reservations are removed. -
Test case:
settables 10x0
/settables 0x10
/settables 0
/settables abc
Expected: No tables set. Error details shown in the result display, with a result messageInvalid Command Format! ...
Checking for reservations
-
Getting the reservations that are for the specified date time, when the date time has at least 1 reservation
- Prerequisites: Date time has at least 1 reservation
- Test case:
check 2021-12-24 2000
Expected: Reservations on 24 Dec 2021, 8pm are displayed, result display shows how many reservations there are on 24 Dec 2021, 8pm
-
Getting the reservations that are for the specified date time, when the date time has no reservations
- Prerequisites: Date time has no reservations
- Test case:
check 2021-12-24 0400
Expected: No Reservations are displayed since no reservations are on 24 Dec 2021, 4am, result display shows 0 reservations
-
Getting the reservations that are for the specified date, when the date has at least 1 reservation
- Prerequisites: Date has at least 1 reservation
- Test case:
check 2021-12-24
Expected: Reservations on 24 Dec 2021 are displayed, result display shows how many reservations there are on 24 Dec 2021
-
Getting the reservations that are for the specified date, when the date has no reservations
- Prerequisites: Date has no reservations
- Test case:
check 2021-12-01
Expected: No Reservations are displayed since no reservations are on 1 Dec 2021, result display shows 0 reservations
-
Getting the reservations that are for the current date at a specified time, when the time has at least 1 reservation
- Prerequisites: Time has at least 1 reservation
- Test case:
check 2000
Expected: Reservations on 24 Dec 2021 are displayed, result display shows how many reservations there are on 24 Dec 2021
-
Getting the reservations that are for the current date at a specified time, when the time has no reservations
- Prerequisites: Time has no reservations
- Test case:
check 0400
Expected: No Reservations are displayed since no reservations are on the current date at 4am, result display shows 0 reservations
-
Other incorrect commands to try:
check 2500
,check abc
,check
Expected: Error details shown in the result display, with a result messageInvalid Command Format! ...
Sorting the customer list
-
Sorting the customer list while all customers are being shown
- Prerequisites: List all customers using
listC
and useresetC
to reset any previous sorting that you may have applied. Note that customers are sorted by name in ascending order by default. - Test case:
sortC by/n o/d
Expected: The customer list is sorted by name in descending order. Success message shown in results display. - Test case:
sortC by/lp o/a
Expected: The customer list is sorted by loyalty points in ascending order. Success message shown in results display. - Test case:
sortC by/n o/a by/lp
Expected: The customer list is sorted by loyalty points in ascending order. Success message shown in results display. - Test case:
sortC by/lp o/a o/d
Expected: The customer list is sorted by loyalty points in descending order. Success message shown in results display. - Test case:
sortC by/lp o/n
Expected: Customer list is not sorted. Error details shown in the result display, with a result messageSorting order can only be either ascending or descending, and it should not be blank
- Test case:
sortC by/dd o/d
Expected: Customer list not sorted. Error details shown in the result display, with a result messageSort by can only be 1 of the customer fields...
- Prerequisites: List all customers using
-
Sorting the customer list while the customer list is filtered.
- Prerequisites: Filter and display the customer list using
findC KEYWORDS
- Test case:
sortC by/n o/d
when filtered customer list isempty
Expected: Customer list is not sorted. Error details shown in the result display, with a result messageCustomer list is currently empty!
- Repeat the same test cases as 1.2 to 1.7. The filtered customer list will sort as per expected in test cases 1.2 to 1.7
- Prerequisites: Filter and display the customer list using
Sorting the employee list
-
Sorting the employee list while all employees are being shown
- Prerequisites: List all employees using
listE
and useresetE
to reset any previous sorting that you may have applied. Note that employees are sorted by name in ascending order by default. - Test case:
sortE by/sal o/a
Expected: The employee list is sorted by salary in ascending order. Success message shown in results display. - Test case:
sortE by/n o/d
Expected: The employee list is sorted by name in descending order. Success message shown in results display. - Test case:
sortE by/n o/a by/sal
Expected: The employee list is sorted by salary in ascending order. Success message shown in results display. - Test case:
sortE by/n o/a o/d
Expected: The employee list is sorted by name in descending order. Success message shown in results display. - Test case:
sortE by/e o/n
Expected: Employee list is not sorted. Error details shown in the result display, with a result messageSorting order can only be either ascending or descending, and it should not be blank
- Test case:
sortE by/dd o/d
Expected: Employee list not sorted. Error details shown in the result display, with a result messageSort by can only be 1 of the employee fields...
- Prerequisites: List all employees using
-
Sorting the employee list while the employee list is filtered.
- Prerequisites: Filter and display the employee list using
findE KEYWORDS
- Test case:
sortE by/n o/d
when filtered employee list isempty
Expected: Employee list is not sorted. Error details shown in the result display, with a result messageEmployee list is currently empty!
- Repeat the same test cases as 1.2 to 1.7. The filtered employee list will sort as per expected in test cases 1.2 to 1.7
- Prerequisites: Filter and display the employee list using
Sorting the Supplier list
-
Sorting the supplier list while all suppliers are being shown
- Prerequisites: List all suppliers using
listS
and useresetS
to reset any previous sorting that you may have applied. Note that suppliers are sorted by name in ascending order by default. - Test case:
sortS by/dd o/d
Expected: The supplier list is sorted by delivery details in descending order. Success message shown in results display. - Test case:
sortS by/st o/a
Expected: The supplier list is sorted by supply type in name order. Success message shown in results display. - Test case:
sortS by/n o/a by/dd
Expected: The supplier list is sorted by delivery details in ascending order. Success message shown in results display. - Test case:
sortS by/n o/a o/d
Expected: The supplier list is sorted by name in descending order. Success message shown in results display. - Test case:
sortS by/a o/n
Expected: Supplier list is not sorted. Error details shown in the result display, with a result messageSorting order can only be either ascending or descending, and it should not be blank
- Test case:
sortS by/lp o/d
Expected: Supplier list not sorted. Error details shown in the result display, with a result messageSort by can only be 1 of the supplier fields...
- Prerequisites: List all suppliers using
-
Sorting the supplier list while the supplier list is filtered.
- Prerequisites: Filter and display the supplier list using
findE KEYWORDS
- Test case:
sortS by/dd o/a
when filtered supplier list isempty
Expected: Supplier list is not sorted. Error details shown in the result display, with a result messageSupplier list is currently empty!
- Repeat the same test cases as 1.2 to 1.7. The filtered supplier list will sort as per expected in test cases 1.2 to 1.7
- Prerequisites: Filter and display the supplier list using
Resetting the customer/employee/supplier
-
Switching the view to display the customer/employee/supplier list to its default sorting order (by
name
inascending
order).- Test case:
resetC
/resetE
/resetS
Expected: List of all customers/employees/suppliers in default ordering will be displayed. Success messageX list sorting has been reset to default (name).
will be displayed (where X refers to eitherCustomer
,Employee
orSupplier
depending on whichreset
command is used). - Test case:
reesetC
/resagE
/ …
Expected: Any form of misspelling will result in no changes to the current view. Error messageUnknown command
will be displayed.
- Test case: